Understanding @State and @Binding in SwiftUI - A Visual Guide
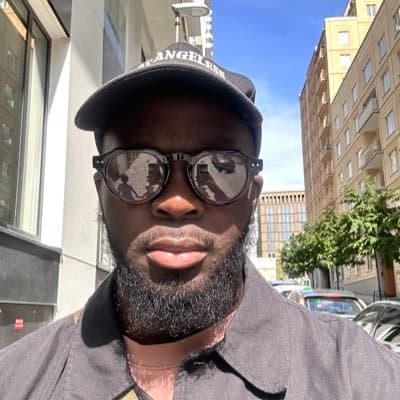
Mansour Mahamat
11/18/2024

As a beginner in SwiftUI, understanding state management is crucial for building interactive applications. In this guide, we'll explore two fundamental property wrappers: @State
and @Binding
. We'll learn how they work and build practical examples together.
What is State Management?
Before diving into the specifics, let's understand what state management means. State in your app is any data that can change over time, like:
- Text in a text field
- Whether a toggle is on or off
- The current score in a game
- Items in a shopping cart
When this data changes, SwiftUI needs to update the user interface to reflect these changes. This is where @State
and @Binding
come in.
Understanding @State
@State
is a property wrapper that tells SwiftUI to manage a value and update the view when that value changes. Think of it as a way to make your view "react" to changes in data.
Let's start with a simple example:
struct CounterView: View {
@State private var count = 0
var body: some View {
VStack {
Text("Count: \(count)")
.font(.title)
Button("Increment") {
count += 1
}
.padding()
}
}
}
In this example:
@State private var count = 0
creates a piece of state- When the button is tapped,
count
updates - SwiftUI automatically refreshes the view to show the new count
Common @State Use Cases
Here are more practical examples of using @State
:
struct UserInputExample: View {
@State private var name = ""
@State private var isSubscribed = false
@State private var selectedColor = "Red"
let colors = ["Red", "Blue", "Green"]
var body: some View {
Form {
// Text Input
TextField("Enter your name", text: $name)
// Toggle
Toggle("Subscribe to newsletter", isOn: $isSubscribed)
// Picker
Picker("Select color", selection: $selectedColor) {
ForEach(colors, id: \.self) { color in
Text(color)
}
}
// Display values
Text("Hello, \(name)!")
Text("Newsletter: \(isSubscribed ? "Yes" : "No")")
Text("Favorite color: \(selectedColor)")
}
}
}
Notice the $
symbol before state variables when using them with SwiftUI controls. This brings us to our next topic: @Binding
.
Understanding @Binding
@Binding
creates a reference to a state variable that's defined somewhere else. It's like a "connection" to a piece of state, allowing child views to modify their parent's state.
Let's create a custom toggle component to understand this:
// Custom toggle component
struct CustomToggle: View {
@Binding var isOn: Bool
let title: String
var body: some View {
Toggle(title, isOn: $isOn)
.tint(.green)
.padding()
}
}
// Parent view using the custom toggle
struct ParentView: View {
@State private var isEnabled = false
var body: some View {
VStack {
CustomToggle(isOn: $isEnabled, title: "Enable Feature")
if isEnabled {
Text("Feature is enabled!")
.foregroundColor(.green)
}
}
}
}
Key points about @Binding
:
- It uses the
$
prefix when passing state to the binding - Changes in either view are reflected in both places
- The parent owns the state, the child just has a reference
Practical Project: Todo List
Let's combine our knowledge to build a simple todo list:
// Todo item model
struct TodoItem: Identifiable {
let id = UUID()
var title: String
var isCompleted: Bool
}
// Todo item row view
struct TodoItemRow: View {
@Binding var item: TodoItem
var body: some View {
HStack {
Text(item.title)
Spacer()
Toggle("", isOn: $item.isCompleted)
}
}
}
// Main todo list view
struct TodoListView: View {
@State private var todos: [TodoItem] = []
@State private var newTodoTitle = ""
var body: some View {
VStack {
// Add new todo
HStack {
TextField("New todo", text: $newTodoTitle)
Button("Add") {
if !newTodoTitle.isEmpty {
todos.append(TodoItem(title: newTodoTitle, isCompleted: false))
newTodoTitle = ""
}
}
}
.padding()
// Todo list
List {
ForEach($todos) { $todo in
TodoItemRow(item: $todo)
}
}
}
}
}
Common Mistakes to Avoid
-
Using @State for Complex Data
@State
is designed for simple values- Use
@StateObject
for complex objects
-
Forgetting the $ Prefix
// Wrong
TextField("Name", text: name)
// Correct
TextField("Name", text: $name)
- Making @State Public
// Wrong
@State public var count = 0
// Correct
@State private var count = 0
Best Practices
-
Keep State at Appropriate Level
- Place state in the highest view that needs access to it
- Pass only what child views need via bindings
-
Use Meaningful Names
// Not descriptive
@State private var x = false
// Better
@State private var isShowingDetail = false
- Initialize State Properties
@State private var score = 0 // Good
@State private var name = "" // Good
@State private var items: [String] // Bad - uninitialized
Next Steps
Now that you understand @State
and @Binding
, you can explore:
@Observable
for reference types (iOS 17+)@Bindable
for reference type properties (iOS 17+)@Environment
for dependency injection@AppStorage
for persistent storage
Conclusion
Understanding state management is fundamental to SwiftUI development. Remember:
- Use
@State
for simple value types that can change - Use
@Binding
to share state with child views - Use
@Observable
for reference types (iOS 17+) - Always make state private when possible
For complex state management in modern SwiftUI apps:
- Prefer the new
@Observable
macro over older property wrappers - Use
@Bindable
when you need two-way bindings with observable types - Consider using the Environment system for dependency injection
These newer patterns introduced in iOS 17 provide a more elegant way to handle complex state compared to older approaches.
Happy coding! 🚀