Using Core Data for Local Data Storage in SwiftUI
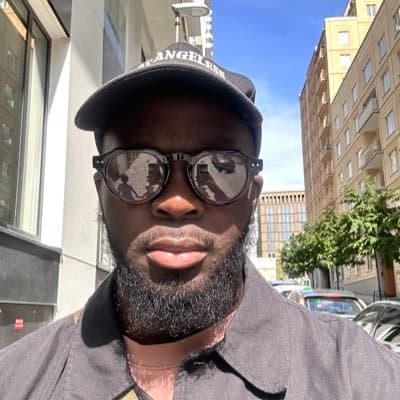
Mansour Mahamat
10/17/2024
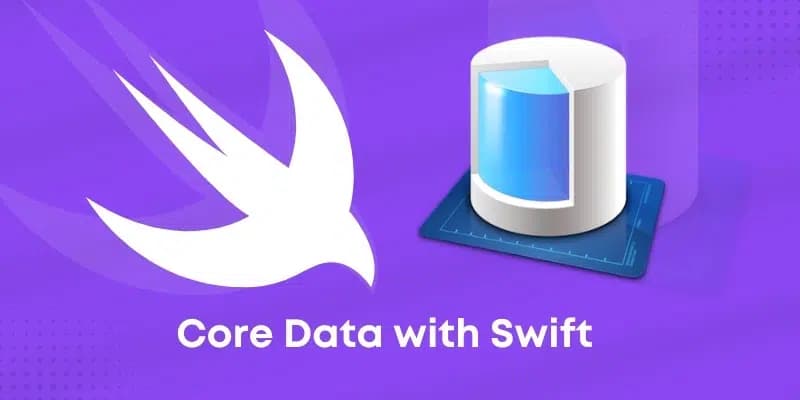
Core Data is a powerful framework provided by Apple for managing and persisting data in iOS applications. When combined with SwiftUI, it offers a robust solution for local data storage. In this beginner-friendly guide, we'll walk through the process of integrating Core Data into a SwiftUI project.
What is Core Data?
Core Data is not a database itself, but rather a framework that manages an object graph and persists data to disk. It provides an abstraction layer between your application's model objects and the underlying data storage, making it easier to work with complex data in your app.
Setting Up Core Data in a SwiftUI Project
Let's start by setting up a new SwiftUI project with Core Data integration:
- Open Xcode and create a new project.
- Choose "App" under the iOS tab.
- Name your project (e.g., "CoreDataDemo").
- Make sure "Use Core Data" is checked before creating the project.
Xcode will generate a project with Core Data already integrated, including a .xcdatamodeld
file for your data model.
Defining Your Data Model
The first step in using Core Data is defining your data model:
- Open the
.xcdatamodeld
file in your project. - Click the "Add Entity" button to create a new entity.
- Name your entity (e.g., "Task").
- Add attributes to your entity (e.g., "title" as String, "isCompleted" as Boolean).
Here's what your data model might look like:
Entity: Task
Attributes:
- title: String
- isCompleted: Boolean
- createdAt: Date
Creating a Managed Object Context
SwiftUI provides a convenient way to access the managed object context through the environment. In your App
struct, you'll see something like this:
@main
struct CoreDataDemoApp: App {
let persistenceController = PersistenceController.shared
var body: some Scene {
WindowGroup {
ContentView()
.environment(\.managedObjectContext, persistenceController.container.viewContext)
}
}
}
This code sets up the managed object context and makes it available to all views in your app.
Creating and Saving Data
Now, let's create a view to add new tasks:
struct AddTaskView: View {
@Environment(\.managedObjectContext) private var viewContext
@Environment(\.dismiss) private var dismiss
@State private var taskTitle = ""
var body: some View {
Form {
TextField("Task title", text: $taskTitle)
Button("Save") {
addTask()
}
}
}
private func addTask() {
let newTask = Task(context: viewContext)
newTask.title = taskTitle
newTask.isCompleted = false
newTask.createdAt = Date()
do {
try viewContext.save()
dismiss()
} catch {
print("Error saving task: \(error)")
}
}
}
This view uses the @Environment
property wrapper to access the managed object context. When the "Save" button is tapped, it creates a new Task
object and saves it to Core Data.
Fetching and Displaying Data
To display the tasks, we'll use SwiftUI's @FetchRequest
property wrapper:
struct TaskListView: View {
@Environment(\.managedObjectContext) private var viewContext
@FetchRequest(
sortDescriptors: [NSSortDescriptor(keyPath: \Task.createdAt, ascending: true)],
animation: .default)
private var tasks: FetchedResults<Task>
var body: some View {
List {
ForEach(tasks) { task in
TaskRow(task: task)
}
.onDelete(perform: deleteTasks)
}
}
private func deleteTasks(offsets: IndexSet) {
withAnimation {
offsets.map { tasks[$0] }.forEach(viewContext.delete)
do {
try viewContext.save()
} catch {
print("Error deleting task: \(error)")
}
}
}
}
struct TaskRow: View {
@ObservedObject var task: Task
var body: some View {
Toggle(isOn: $task.isCompleted) {
Text(task.title ?? "")
}
.onChange(of: task.isCompleted) { _ in
try? task.managedObjectContext?.save()
}
}
}
This view uses @FetchRequest
to retrieve all tasks from Core Data, sorted by creation date. It displays each task as a toggle, allowing the user to mark tasks as completed.
Updating Data
In the TaskRow
view, we use @ObservedObject
to observe changes to the task. When the toggle is switched, the onChange
modifier saves the updated state to Core Data.
Putting It All Together
Finally, let's create a main view that combines the task list and the ability to add new tasks:
struct ContentView: View {
@State private var showingAddTask = false
var body: some View {
NavigationView {
TaskListView()
.navigationTitle("Tasks")
.toolbar {
Button(action: { showingAddTask = true }) {
Label("Add Task", systemImage: "plus")
}
}
.sheet(isPresented: $showingAddTask) {
AddTaskView()
}
}
}
}
This view presents the TaskListView
and provides a button to show the AddTaskView
as a sheet.
Conclusion
We've covered the basics of using Core Data with SwiftUI:
- Setting up Core Data in a SwiftUI project
- Defining a data model
- Creating and saving data
- Fetching and displaying data
- Updating and deleting data
Core Data provides a powerful way to manage local data in your iOS apps. As you become more comfortable with these concepts, you can explore more advanced features like relationships between entities, data migration, and iCloud sync.
Remember to handle errors gracefully in a production app and consider the user experience when dealing with data operations. Happy coding!